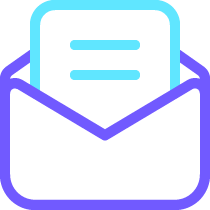
Swift // Array to keep track of controllers in page menu var controllerArray : [UIViewController] = [] // Create variables for all view controllers you want to put in the // page menu, initialize them, and add each to the controller array.
Full Answer
How to create a sidebar menu in Swift?
In Swift, you just need to specify the method name as a string literal to construct a selector. Lastly, we add a gesture recognizer. Not only you can use the menu button to bring out the sidebar menu, the user can swipe the content area to activate the sidebar as well. Cool! Let’s compile and run the app in the simulator.
How to use the menu in the navigation bar?
User triggers the menu by tapping the list button at the top-left of navigation bar. User can also bring up the menu by swiping right on the main content area. Once the menu appears, user can close it by tapping the list button again.
How to create a sidebar menu using swrevealviewcontroller?
To use SWRevealViewController for building a sidebar menu, you create a container view controller, which is actually an empty view controller, to hold both the menu view controller and a set of content view controllers. I have already created the menu view controller for you. It is just a static table view with three menu items.
How to create sidebar menu in WordPress?
Normally, the navigation menu is hidden behind the front view. The menu can then be triggered by tapping a list button in the navigation bar. Once the menu is expanded and becomes visible, users can close it by using the list button or simply swiping left on the content area. You can build the sidebar menu from the ground up.

Overview
The following example presents a menu of three buttons and a submenu, which contains three buttons of its own.
Primary Action
Menus can be created with a custom primary action. The primary action will be performed when the user taps or clicks on the body of the control, and the menu presentation will happen on a secondary gesture, such as on long press or on click of the menu indicator.
Styling Menus
Use the menuStyle (_:) modifier to change the style of all menus in a view. The following example shows how to apply a custom style:
PagingMenuControllerCustomizable
isScrollEnabled for paging view. Set false in case of using swipe-to-delete on your table view
Using Storyboard
struct MenuItem1: MenuItemViewCustomizable {} struct MenuItem2: MenuItemViewCustomizable {} struct MenuOptions: MenuViewCustomizable { var itemsOptions: [MenuItemViewCustomizable] { return [MenuItem1 (), MenuItem2 ()] } } struct PagingMenuOptions: PagingMenuControllerCustomizable { var componentType: ComponentType { return .all (menuOptions: MenuOptions (), pagingControllers: [UIViewController (), UIViewController ()]) } } let pagingMenuController = self.childViewControllers.first as! PagingMenuController pagingMenuController.setup (options) pagingMenuController.onMove = { state in switch state { case let .willMoveController (menuController, previousMenuController): print (previousMenuController) print (menuController) case let .didMoveController (menuController, previousMenuController): print (previousMenuController) print (menuController) case let .willMoveItem (menuItemView, previousMenuItemView): print (previousMenuItemView) print (menuItemView) case let .didMoveItem (menuItemView, previousMenuItemView): print (previousMenuItemView) print (menuItemView) case .didScrollStart: print ("Scroll start") case .didScrollEnd: print ("Scroll end") } }.
Coding only
struct MenuItem1: MenuItemViewCustomizable {} struct MenuItem2: MenuItemViewCustomizable {} struct MenuOptions: MenuViewCustomizable { var itemsOptions: [MenuItemViewCustomizable] { return [MenuItem1 (), MenuItem2 ()] } } struct PagingMenuOptions: PagingMenuControllerCustomizable { var componentType: ComponentType { return .all (menuOptions: MenuOptions (), pagingControllers: [UIViewController (), UIViewController ()]) } } let options = PagingMenuOptions () let pagingMenuController = PagingMenuController (options: options) addChildViewController (pagingMenuController) view.addSubview (pagingMenuController.view) pagingMenuController.didMove (toParentViewController: self).
Menu move handler (optional)
public enum MenuMoveState { case willMoveController (to: UIViewController, from: UIViewController) case didMoveController (to: UIViewController, from: UIViewController) case willMoveItem (to: MenuItemView, from: MenuItemView) case didMoveItem (to: MenuItemView, from: MenuItemView) case didScrollStart case didScrollEnd } pagingMenuController.onMove = { state in switch state { case let .willMoveController (menuController, previousMenuController): print (previousMenuController) print (menuController) case let .didMoveController (menuController, previousMenuController): print (previousMenuController) print (menuController) case let .willMoveItem (menuItemView, previousMenuItemView): print (previousMenuItemView) print (menuItemView) case let .didMoveItem (menuItemView, previousMenuItemView): print (previousMenuItemView) print (menuItemView) case .didScrollStart: print ("Scroll start") case .didScrollEnd: print ("Scroll end") } }.
Changing PagingMenuController's option
Call setup method with new options again. It creates a new paging menu controller. Do not forget to cleanup properties in child view controller.
CocoaPods
PagingMenuController is available through CocoaPods. To install it, simply add the following line to your Podfile:
Description
A fully customizable and flexible paging menu controller built from other view controllers placed inside a scroll view allowing the user to switch between any kind of view controller with an easy tap or swipe gesture similar to what Spotify, Windows Phone, and Instagram use
How to use PageMenu
First you will have to create a view controller that is supposed to serve as the base of the page menu. This can be a view controller with its xib file as a separate file as well as having its xib file in storyboard. Following this you will have to go through a few simple steps outlined below in order to get everything up and running.
Customization
There are many ways you are able to customize page menu for your needs and there will be more customizations coming in the future to make sure page menu conforms to your app design. These will all be properties in CAPSPageMenu that can be changed from your base view controller. (Property names given with each item below)
Apps using PageMenu
Please let me know if your app in the AppStore uses this library so I can add your app to the list of apps featuring PageMenu.
License
Copyright (c) 2014 The Board of Trustees of The University of Alabama All rights reserved.
How to use SWRevealViewController?
To use SWRevealViewController for building a sidebar menu, you create a container view controller, which is actually an empty view controller, to hold both the menu view controller and a set of content view controllers. I have already created the menu view controller for you.
What is the target action mechanism in Cocoa?
As you know, Cocoa uses the target-action mechanism for communication between a control and another object. We set the target of the menu button to the reveal view controller and action to the revealToggle: method. So when the menu button is tapped, it will call the revealToggle: method to display the sidebar menu.
What is the front view controller?
The front view controller is the main controller for displaying content. In our storyboard, it’s the navigation controller which associates with a view controller for presenting news. The rear view controller is the controller that shows the navigation menu. Here it is the Sidebar View Controller. Go to the storyboard.
How many content view controllers are there?
There are three content view controllers for displaying news, map and photos. For demo purpose, the content view controllers only shows static data. And I just created three controllers. If you need to have a few more controllers, simply insert them into the storyboard.
ModularSidebarView
ModularSidebarView is a customizable menu for displaying options on the side of the screen for iOS. It is meant to act as a substitute to the usual UINavigation bar items and tool bar items.
Usage
Then create some sort of UIButton, UIBarButtonItem or any view with userInteraction enabled. Create the selector and function however you choose.
Adding items to the SidebarView
You may subclass the default provided classes or conform to the underlying protocol for more customization
Example
To run the example project, clone the repo, and run pod install from the Example directory first.
Installation
ModularSidebarView is available through CocoaPods. To install it, simply add the following line to your Podfile:
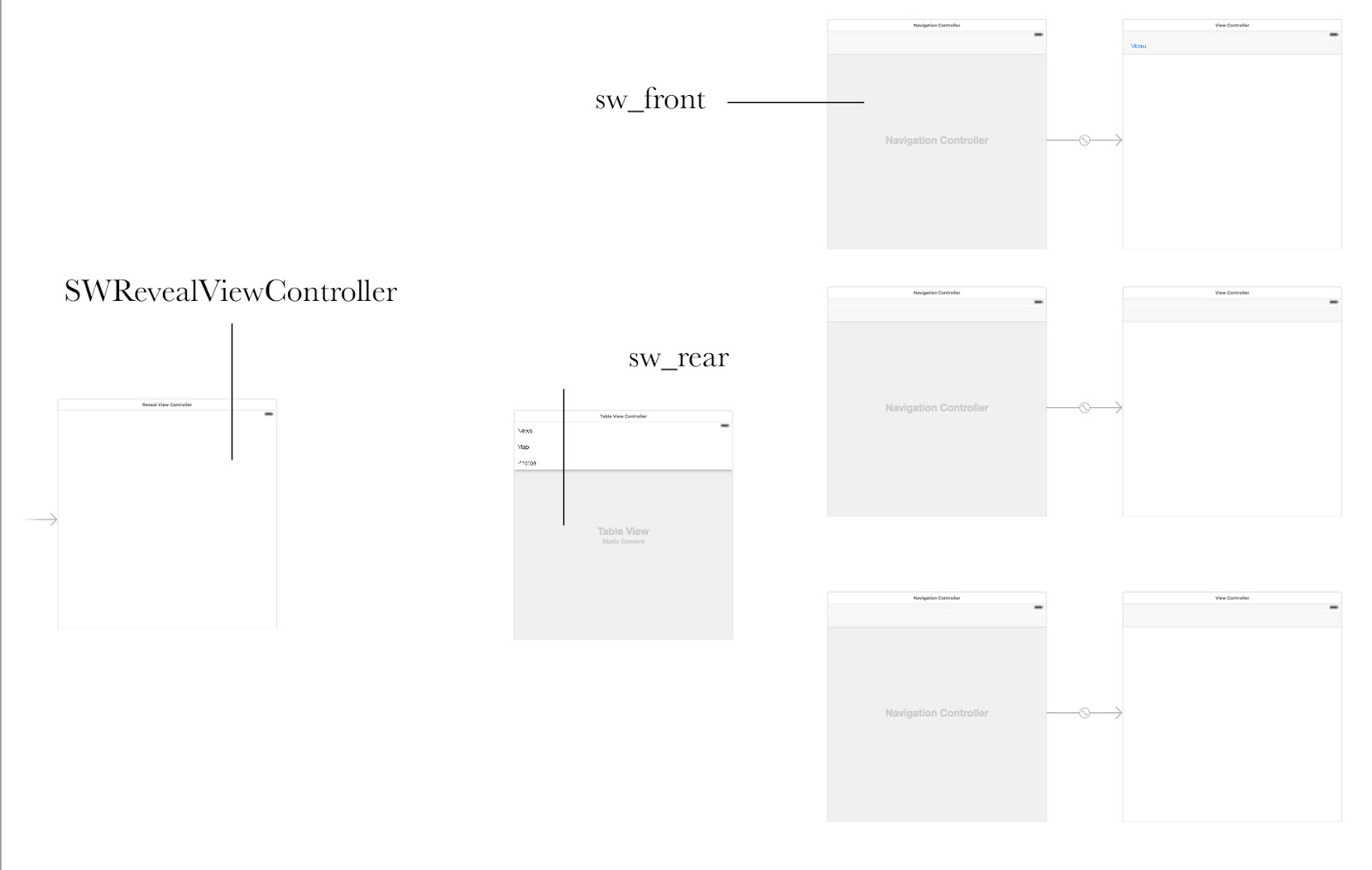
Description
Installation
- CocoaPods PageMenu is available through CocoaPods. !! Swift only !! To install add the following line to your Podfile: Carthage PageMenu is also available through Carthage. Append this line to Cartfile and follow this instruction. Manual Installation The class file required for PageMenu is located in the Classes folder in the root of this repository as listed below: 1. CAPSPageMenu.swift
How to Use PageMenu
- First you will have to create a view controller that is supposed to serve as the base of the page menu. This can be a view controller with its xib file as a separate file as well as having its xib file in storyboard. Following this you will have to go through a few simple steps outlined below in order to get everything up and running. 1) Add the files listed in the installation section to your pr…
Customization
- There are many ways you are able to customize page menu for your needs and there will be more customizations coming in the future to make sure page menu conforms to your app design. These will all be properties in CAPSPageMenu that can be changed from your base view controller. (Property names given with each item below) 1) Colors 1. Background color behind th…
Apps Using PageMenu
- Please let me know if your app in the AppStore uses this library so I can add your app to the list of apps featuring PageMenu.
Future Work
- Screen rotation support
- Objective-C version
- Infinite scroll option / Wrap items
- Carthage support
Credits
- Niklas Fahl (fahlout) - iOS Developer (LinkedIn) Thank you for your contributions: masarusanjp 1. Type-safe options John C. Daub (hsoi) 1. iOS 7.1 fixes 2. Content size fixes on viewDidLayoutSubviews() Gurpartap Singh (Gurpartap) 1. CocoaPods fixes 2. ScrollToTop fixes Chao Ruan (rcgary) 1. Swift 1.2 Support
Update Log
- 1.2.7 Release (06/05/2015) 1. CocoaPods now has current version 2. Objective-C version in Beta 3. Demos updated 1.2.6 Release (05/26/2015) 1. Options are now type-safe - Thanks to masarusanjp 1.2.5 Release (04/14/2015) 1. Support for Swift 1.2 - Thanks to Chao Ruan (rcgary) 2. Will be on cocoa pods soon! 1.2.4 Release (03/24/2015) 1. Small improvements thanks to hso…
License
- Copyright (c) 2014 The Board of Trustees of The University of AlabamaAll rights reserved. Redistribution and use in source and binary forms, with or withoutmodification, are permitted provided that the following conditionsare met: 1. Redistributions of source code must retain the above copyrightnotice, this list of conditions and the following disclaimer. 2. Redistributions in b…